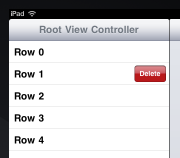
this is just a quick tutorial for those like me who didn't implement this before. Luckily this is one of the nice UI features that can be implemented without much hassle.
You only have to do a few things to get this working.
1) Tell your
UITableViewDelegate
that your rows have a delete editing style:- (UITableViewCellEditingStyle) tableView:(UITableView*)tableView editingStyleForRowAtIndexPath:(NSIndexPath*)indexPath {
return UITableViewCellEditingStyleDelete;
}
2) Implement
commitEditingStyle:
in your UITableViewDataSource
. In order to get the delete button displayed it is enough to just have the function implemented without doing anything:- (void)tableView:(UITableView*)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath*)indexPath {
// delete your data for this row from here
}
3) Now if you actually want to delete that row visually you have to add more to
commitEditingStyle
. And before you do that visual delete, you have to change your data beforehand so thatnumberOfRowsInSection:
returns less rows than before:- (NSInteger)tableView:(UITableView*)aTableView numberOfRowsInSection:(NSInteger)section {
return numRows;
}
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath*)indexPath {
numRows--;
[self.tableView deleteRowsAtIndexPaths:[NSArray arrayWithObject:indexPath] withRowAnimation:UITableViewRowAnimationFade];
}
And that's it!
No comments:
Post a Comment